Integrating workforce management software into existing business applications can be a challenging process. However, with 7shifts’s API and Konfig’s TypeScript SDK, developers can effortlessly streamline the connection between various systems, reducing both development time and complexity.
Why Use 7shifts’s API?
7shifts is a leading workforce management platform designed to help restaurants optimize labor costs, streamline scheduling, and improve overall team communication. By leveraging 7shifts’s API, developers can programmatically access crucial data, including:
- Employee schedules and shifts
- Time tracking and attendance
- Labor cost analytics
- Task assignments
- Compliance and payroll information
The API enables seamless integration into external applications, allowing businesses to enhance their workforce operations while maintaining flexibility.
The Power of Konfig’s TypeScript SDK
Manually managing API integrations can be time-consuming and prone to errors. This is where Konfig’s TypeScript SDK proves invaluable. The SDK simplifies interactions with the 7shifts API by providing prebuilt functions, strong TypeScript typings, and a user-friendly interface. Some key advantages of using Konfig’s SDK include:
- Automatic type definitions for all API responses
- Built-in authentication handling
- Reduced need to write boilerplate code
- Enhanced error handling mechanisms
- Speedy development by leveraging reusable methods
Steps to Integrate 7shifts’s API with Konfig’s TypeScript SDK
Getting started with 7shifts’s API using Konfig’s SDK is a straightforward process. Below are the essential steps:
1. Install the SDK
First, install the Konfig TypeScript SDK using npm:
npm install @konfig/7shifts-sdk
This will add the necessary dependency to your project.
2. Initialize the SDK
Set up the SDK by importing the module and configuring API credentials:
import { SevenShiftsClient } from '@konfig/7shifts-sdk';
const client = new SevenShiftsClient({
apiKey: 'YOUR_7SHIFTS_API_KEY'
});
Replace YOUR_7SHIFTS_API_KEY
with your actual API key to authenticate requests.
3. Fetch Employee Data
Retrieve a list of employees using a simple method:
async function fetchEmployees() {
try {
const employees = await client.getEmployees();
console.log(employees);
} catch (error) {
console.error('Error fetching employees:', error);
}
}
fetchEmployees();
This method fetches employee details without needing to manually construct API requests.
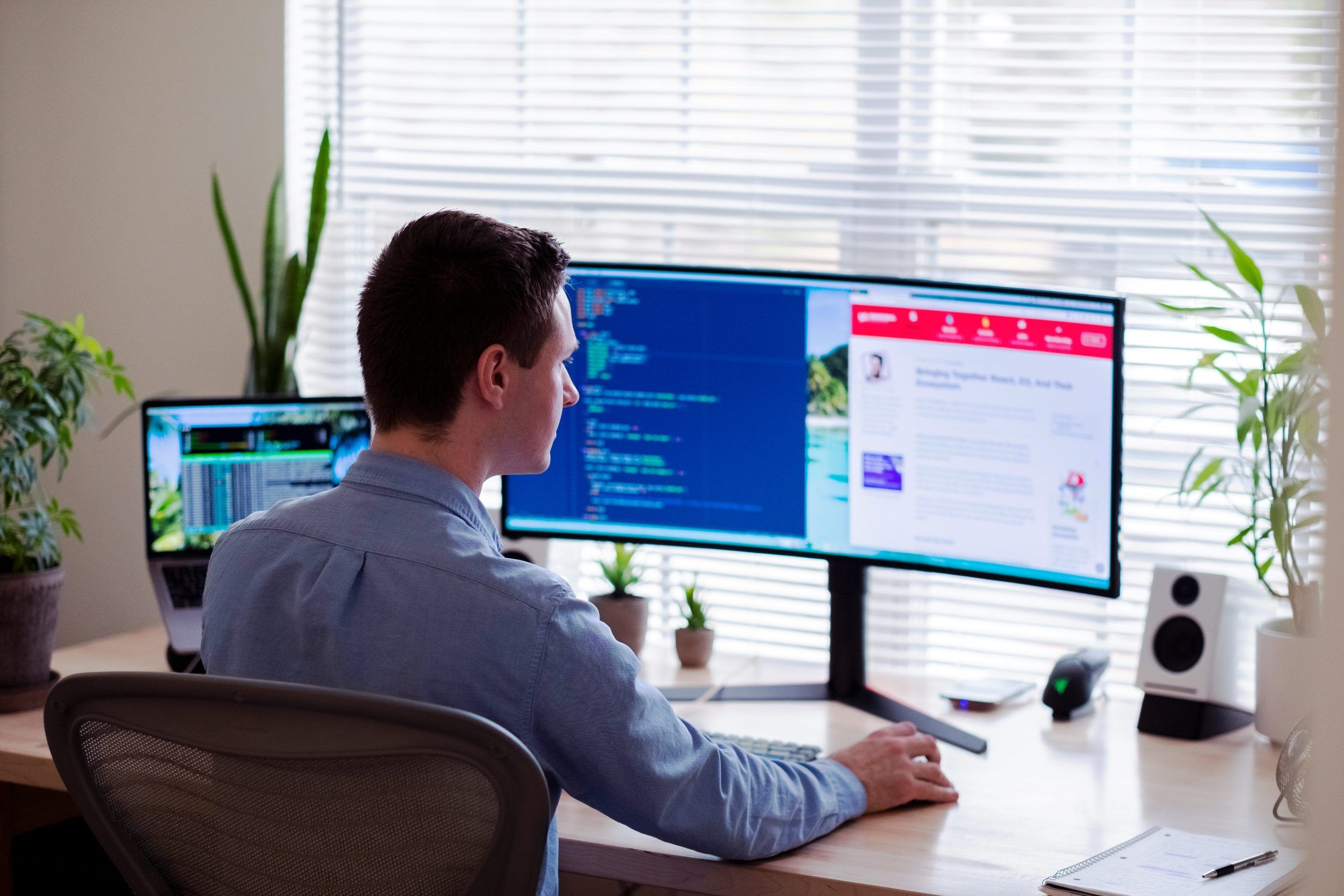
4. Create a New Shift
Adding a shift for an employee is just as simple:
async function createShift() {
try {
const newShift = await client.createShift({
employeeId: 12345,
locationId: 98765,
startTime: '2024-06-01T09:00:00Z',
endTime: '2024-06-01T17:00:00Z'
});
console.log('Shift created:', newShift);
} catch (error) {
console.error('Error creating shift:', error);
}
}
createShift();
By using Konfig’s SDK, developers can ensure smooth API interactions with minimal effort.
Benefits of Using Konfig’s TypeScript SDK
By leveraging Konfig’s TypeScript SDK for 7shifts API integration, businesses and developers gain several key benefits:
- Faster Development: Ready-to-use methods significantly reduce time spent implementing common API interactions.
- Enhanced Stability: The SDK abstracts complex API calls, making applications more robust.
- Better Maintainability: Strong typing and clear method definitions minimize bugs and improve code readability.
- Seamless Authentication: Built-in authentication handling means developers don’t have to manage credentials manually.

Frequently Asked Questions (FAQ)
1. Is the Konfig SDK open-source?
No, Konfig’s TypeScript SDK is a proprietary solution designed to simplify API interactions with 7shifts.
2. What authentication methods does the SDK support?
The SDK primarily supports API key authentication, making it easy to integrate securely.
3. Can I use this SDK with other programming languages?
No, the Konfig SDK is specifically built for use with TypeScript and JavaScript-based applications.
4. How frequently is the SDK updated?
The SDK is regularly updated to align with 7shifts’s API changes and improvements.
5. Does Konfig offer support for integrating the SDK?
Yes, Konfig provides documentation and customer support to assist with implementing the SDK successfully.
6. Can I use this SDK for mobile applications?
Yes, the Konfig TypeScript SDK can be used in both backend and frontend applications, including web and mobile apps.
Final Thoughts
Integrating workforce data through 7shifts’s API is now easier than ever with the power of Konfig’s TypeScript SDK. By leveraging this SDK, businesses can streamline development, reduce errors, and create powerful applications that enhance workforce management. Whether it’s fetching employee data, adding shifts, or processing payroll, the SDK simplifies these processes, enabling developers to focus on building better solutions.